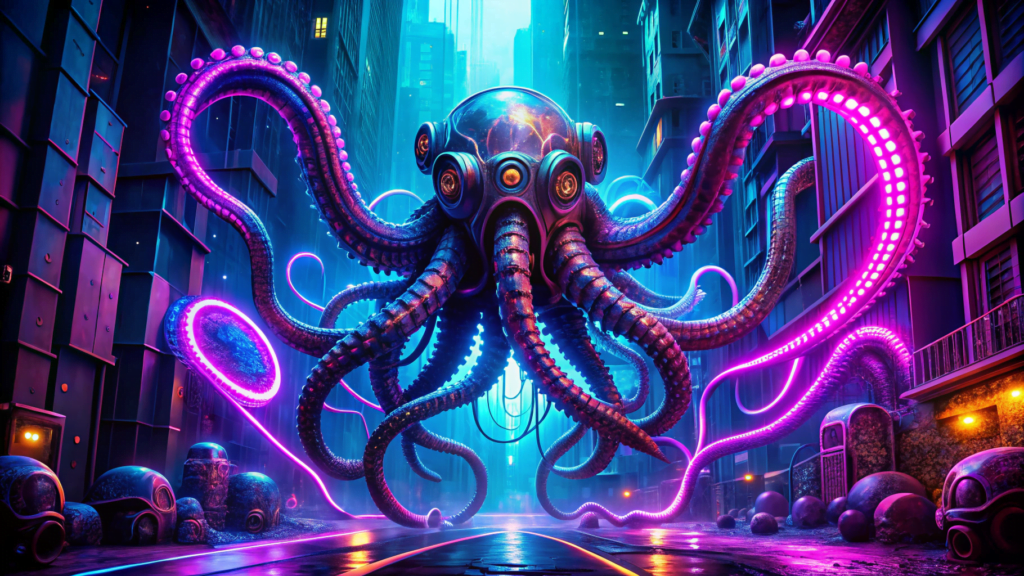
PROJECT
Download Sorter
Project Overview
Downloads are by far the most unruly and prone-to-chaos location on a computer. A close second being the desktop and the third place is My Documents; in my opinion, this is the case, anyway.
It’s a very entry-level project in terms of programming, but it’s an effective one and worth doing.
In this micro-project, I’ll be making an automatic download sorter.
Platform
This script is going to be written in Python. If you haven’t installed Python, then do so through the Microsoft store (it’s free). You’ll then be able to run Python code. Next, you need a way to write that code. You can download and install VSCode, or you can use something basic like Notepad++.
For this, I’ll be using Notepad++ because if you’re following along and are new to Python, you might as well start out small. You can use VSCode for another project but if not, it doesn’t matter; the code is the same.
Notepad++: https://notepad-plus-plus.org/downloads/
VSCode: https://code.visualstudio.com
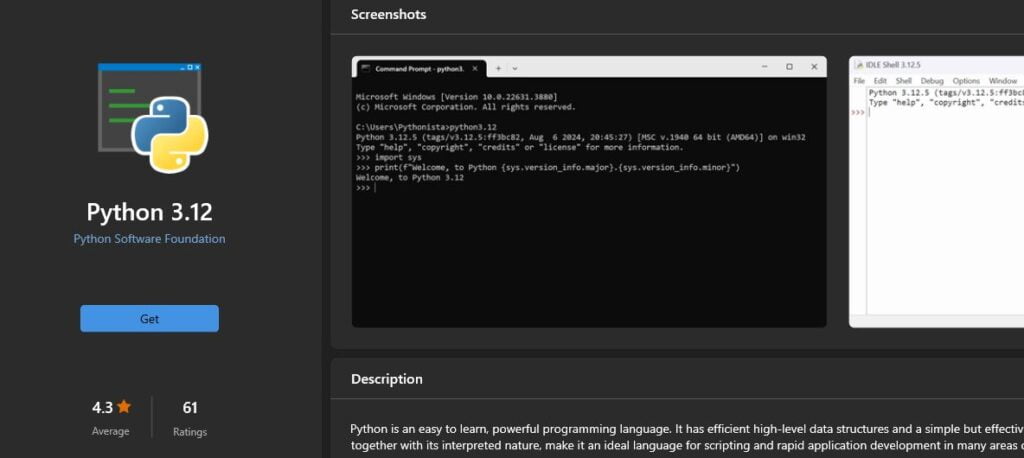
Script
Firstly, create a new file and name it as you like; I’ll be using download_sorter.py. Make sure to set the file extension as .py so it becomes a python file.
Part 1: Scan for files
Firstly, we need to tell Python to import the OS, tell it where the downloads folder is, and tell it to find all of the files. The ‘r’ before the folder path turns it into a raw string, meaning the backslashes don’t act as escapes; if you didn’t do this, then C:\path\to\something would be turned into C:pathtosomething. If you didn’t use this method, you would have to double up on backslashes, such as: C:\\path\\to\\your\\folder.
Lastly in this section, I use a list comprehension to loop through all of the items in the list “scanned_files ” and add them to the new list “files” only if those items are of the type: file. This removes any directories in the list.
Part 2: Loop through the files and move them
Next, we have to loop through the files one at a time, find the file extension and move the files into a folder according to the file extension.
The extension string has a lot going on so I’ll break it down a bit.
str() casts the contents of the brackets as a string. As well as enforcing the data type, this allows us to use string methods such as .split in a code editor like VSCode through the auto-complete code writing options.
file.name takes the string ‘file’ which is the current item in the loop (each file), and .name takes the file name only of the full path. We’re taking the text value that is the filename (filename.extension) and then splitting it into a list for each occurrence of a full stop. This will give us a list that might look like this: [ filename, extension ].
Finally, [-1] returns the final item in a list; in this case it’s the file extension without the full stop.
After all that, we check if the folder variable above + \ + file_extension exists, and if not, we make it.
Then we say “if the file doesn’t contain the folder string \ extension, then move it”. This prevents us from moving files that have already been sorted.
Summary
That’s all there is to this one. It runs on start up and organises loose files.
Hopefully this both helps you keep your PC organised and helps you learn a bit about Python.
Below is what the full script should look like.